Best Website Design using HTML and CSS
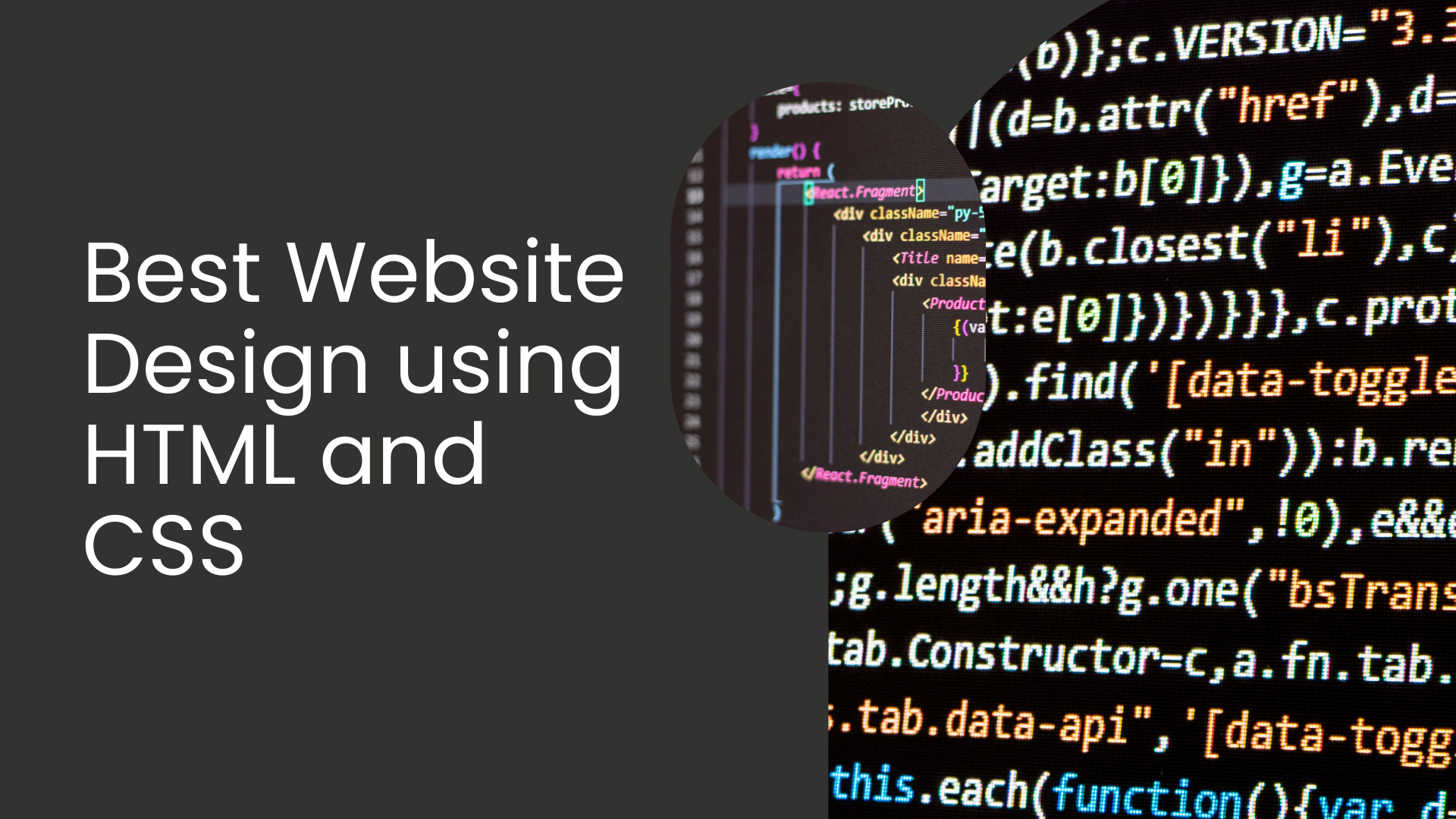
Developing an exemplary website using HTML and CSS necessitates a comprehensive understanding of both technologies, alongside adherence to best practices in usability, accessibility, responsiveness, and aesthetics.
This article delves into the intricacies of HTML and CSS, modern design methodologies, principles of responsive web development, and the frameworks and tools that streamline the design process.
Fundamentals of HTML and CSS
HTML (Hypertext Markup Language) provides the structural foundation for web pages, defining their content and semantic organization. CSS (Cascading Style Sheets) enhances HTML by dictating the visual presentation, encompassing layout, typography, color schemes, and interactive elements.
Core Characteristics of HTML
- Establishes a document structure through semantic elements such as
<header>
,<section>
,<article>
, and<footer>
. - Facilitates multimedia integration with
<img>
,<video>
, and<audio>
elements. - Enables hypertext navigation using
<a>
elements.
Core Characteristics of CSS
- Implements styling rules via selectors, properties, and values.
- Provides sophisticated layout mechanisms, including
flexbox
andgrid
. - Ensures adaptability to various screen dimensions using media queries.
Best Practices in HTML Development
Adhering to structured HTML practices fosters maintainability, scalability, and accessibility.
1. Doctype Declaration
A well-formed HTML document commences with a <!DOCTYPE html>
declaration, ensuring correct interpretation by web browsers.
2. Semantic Markup
Employing semantic elements (<nav>
, <main>
, <aside>
, <footer>
) enhances readability, search engine optimization (SEO), and accessibility.
3. Content-Presentation Separation
Inline styling should be avoided in favor of external CSS files. Example:
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1 class="title">Welcome</h1>
</body>
</html>
.title {
color: navy;
font-size: 24px;
}
4. Logical Code Structuring
Employing <div>
elements judiciously or leveraging appropriate semantic tags facilitates maintainable and modular code architecture.
Best Practices in CSS Development
Optimizing CSS enhances performance and maintainability.
1. Minification and Optimization
Reducing redundant spaces and comments via minification improves load times. Tools such as CSSNano automate this process.
2. Flexbox and Grid for Layout Management
Flexbox and CSS Grid provide dynamic and responsive layout solutions:
.container {
display: flex;
justify-content: space-between;
}
.grid-layout {
display: grid;
grid-template-columns: repeat(3, 1fr);
}
3. Responsive Design via Media Queries
Adaptive styling ensures usability across devices:
@media (max-width: 768px) {
body {
font-size: 14px;
}
}
4. Image Optimization
The <picture>
element and srcset
attribute facilitate responsive image loading.
Responsive Web Design Principles
Effective responsive design incorporates:
- Fluid Grids—Relative units (%, em, rem) supersede fixed pixel values.
- Media Queries—CSS conditions adjust layouts dynamically.
- Mobile-First Approach—Prioritization of mobile optimization before desktop enhancements.
Viewport Meta Tag—Ensures appropriate scaling on mobile devices:
<meta name="viewport" content="width=device-width, initial-scale=1.0">
Frameworks for Efficient Styling
CSS frameworks expedite development through predefined styles and responsive grids.
Notable Frameworks:
- Bootstrap—Comprehensive utility for responsive design.
- Bulma—Lightweight Flexbox-based framework.
- Materialize—Implements Google’s Material Design principles.
Website Development Workflow
1. HTML Structure Definition
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<header>
<h1>Website Title</h1>
</header>
<nav>
<ul>
<li><a href="#about">About</a></li>
<li><a href="#services">Services</a></li>
</ul>
</nav>
<section id="about">
<h2>About Us</h2>
<p>We specialize in modern web solutions.</p>
</section>
</body>
</html>
2. Styling with CSS
body {
font-family: 'Arial', sans-serif;
}
nav ul {
display: flex;
list-style-type: none;
}
nav ul li {
margin-right: 15px;
}
Advanced Techniques in Web Development
1. CSS Animations
Smooth transitions enhance user experience:
button:hover {
background-color: navy;
transition: background-color 0.3s ease-in-out;
}
2. Implementing Dark Mode
Leveraging CSS variables facilitates theme switching:
:root {
--bg-color: white;
--text-color: black;
}
.dark-mode {
--bg-color: black;
--text-color: white;
}
Conclusion
A robust understanding of HTML and CSS is integral to developing responsive, accessible, and aesthetically refined websites. By adhering to best practices, leveraging modern layout techniques, and utilizing frameworks efficiently, developers can create high-performance web applications optimized for diverse user experiences.