C# Project Ideas for Final Year
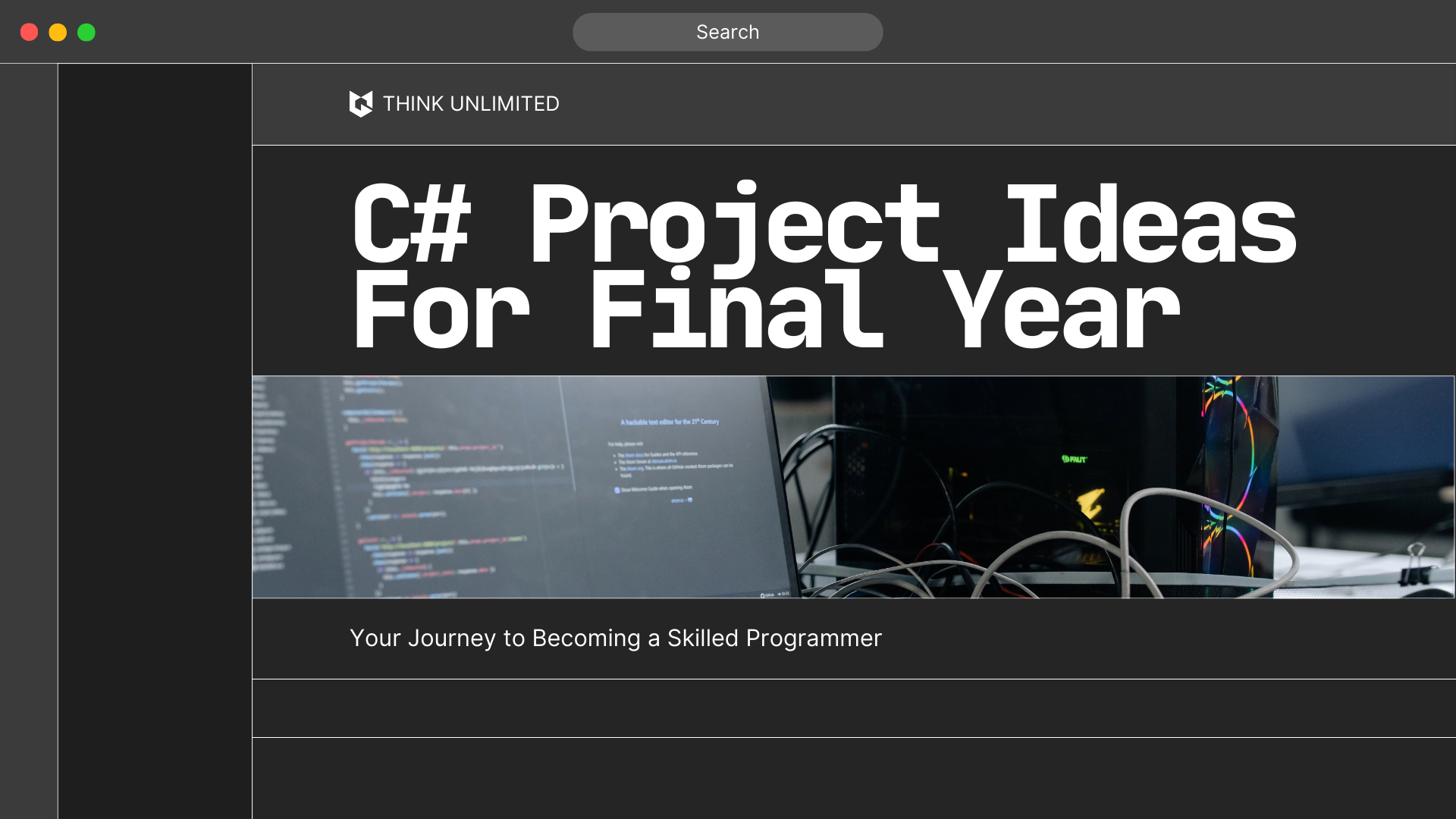
C# is a sophisticated, multi-paradigm programming language extensively utilized in enterprise software development, web-based applications, and game development.
Selecting an appropriate final-year project is pivotal, as it facilitates the consolidation of theoretical knowledge through practical application while enhancing career readiness.
This article categorizes C# project ideas into three tiers—beginner, intermediate, and advanced—ensuring alignment with varying competency levels and professional aspirations.
The Merits of C# for Capstone Projects
C# is an object-oriented language developed by Microsoft within the .NET ecosystem. Its advantages include:
- Syntax Clarity: The language exhibits a structured and readable syntax, mitigating entry barriers for novices.
- Multiparadigm Support: It seamlessly integrates object-oriented, imperative, and functional programming paradigms.
- Extensive Applicability: C# underpins a broad spectrum of development domains, from desktop and cloud-based applications to game development via Unity.
- Industry Relevance: As a consistently high-ranking language in industry adoption metrics, C# proficiency enhances career prospects in software engineering and related disciplines.
Beginner-Level Projects
These projects introduce foundational programming principles such as event-driven programming, data persistence, and user interface interactions.
1. To-Do List Manager
- Objective: Develop a task management application with fundamental CRUD operations.
- Features:
- Task creation, modification, and deletion.
- Status updates (completed/pending).
- Priority-based task categorization.
- Technologies: Visual Studio, .NET Framework, SQLite/JSON for data persistence.
- Conceptual Takeaways:
- Event-driven architecture.
- Persistent data handling.
Code Implementation:
List<string> tasks = new List<string>();
void AddTask(string task) {
tasks.Add(task);
Console.WriteLine("Task Added: " + task);
}
2. Weather App
- Objective: Develop an API-driven application for retrieving real-time meteorological data.
- Features:
- Fetch weather details via an external API.
- Display temperature, humidity, and forecasting insights.
- Implement geolocation-based search capabilities.
- Technologies: .NET Framework, HTTP client libraries for API integration.
- Conceptual Takeaways:
- RESTful API interaction.
- JSON/XML data parsing.
Code Implementation:
using System.Net.Http;
using System.Threading.Tasks;
async Task<string> GetWeatherAsync(string city) {
string apiUrl = $"https://api.openweathermap.org/data/2.5/weather?q={city}&appid=YOUR_API_KEY";
HttpClient client = new HttpClient();
HttpResponseMessage response = await client.GetAsync(apiUrl);
return await response.Content.ReadAsStringAsync();
}
Intermediate-Level Projects
These projects demand proficiency in database integration, user authentication, and software design patterns.
3. Blogging Platform
- Objective: Develop a content management system for blog creation and interaction.
- Features:
- User authentication and session management.
- WYSIWYG editor for post composition.
- Commenting functionality.
- Technologies: ASP.NET Core, SQL Server for data management.
- Conceptual Takeaways:
- MVC (Model-View-Controller) architecture.
- Secure authentication mechanisms.
Code Implementation:
public class BlogPost {
public int Id { get; set; }
public string Title { get; set; }
public string Content { get; set; }
public DateTime DatePosted { get; set; } = DateTime.Now;
}
4. Car Racing Game
- Objective: Construct a rudimentary 2D car racing game with obstacle avoidance and scoring mechanisms.
- Features:
- Keyboard-controlled navigation.
- Randomly spawned obstacles.
- Multi-tier difficulty settings.
- Technologies: WinForms/WPF, GDI+ for graphical rendering.
- Conceptual Takeaways:
- Event-driven game mechanics.
- Collision detection techniques.
Code Implementation:
private void MoveCar(object sender, KeyEventArgs e) {
if (e.KeyCode == Keys.Left) car.Left -= 5;
if (e.KeyCode == Keys.Right) car.Left += 5;
}
Advanced-Level Projects
These projects integrate enterprise-grade design considerations, including concurrency, security, and scalability.
5. Library Management System
- Objective: Develop an automated library system for tracking book circulation and membership records.
- Features:
- Catalog search and categorization.
- Loan and return processing.
- Overdue tracking and reporting.
- Technologies: SQL Server, Entity Framework, WPF for GUI design.
- Conceptual Takeaways:
- Relational database normalization.
- Object-relational mapping (ORM).
Code Implementation:
public class Book {
public int Id { get; set; }
public string Title { get; set; }
public string Author { get; set; }
public bool IsAvailable { get; set; } = true;
}
6. E-Commerce Platform
- Objective: Engineer an online shopping system incorporating secure payment processing and inventory management.
- Features:
- User authentication and order processing.
- Product catalog with search and filter options.
- Payment gateway integration.
- Technologies: ASP.NET Core, SQL Server, JavaScript frameworks (React/Angular).
- Conceptual Takeaways:
- Full-stack application design.
- Secure transaction implementation.
Code Implementation:
public class Product {
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
public string Description { get; set; }
}
Project Selection Considerations
- Skill Alignment: Choose a project commensurate with your technical proficiency.
- Domain Relevance: Tailor the project towards prospective career trajectories, such as web development, game design, or enterprise software engineering.
- Scalability: Opt for projects with potential extensibility to accommodate future refinements or expansions.
Conclusion
Final-year projects constitute a crucial component of academic and professional development. Whether constructing a fundamental task manager or architecting an enterprise-grade application, these C# project ideas equip students with the requisite skills to tackle real-world software challenges.
By engaging with these projects, aspiring developers cultivate expertise in software engineering principles, problem-solving strategies, and contemporary development methodologies.