How to add MCP to any React App
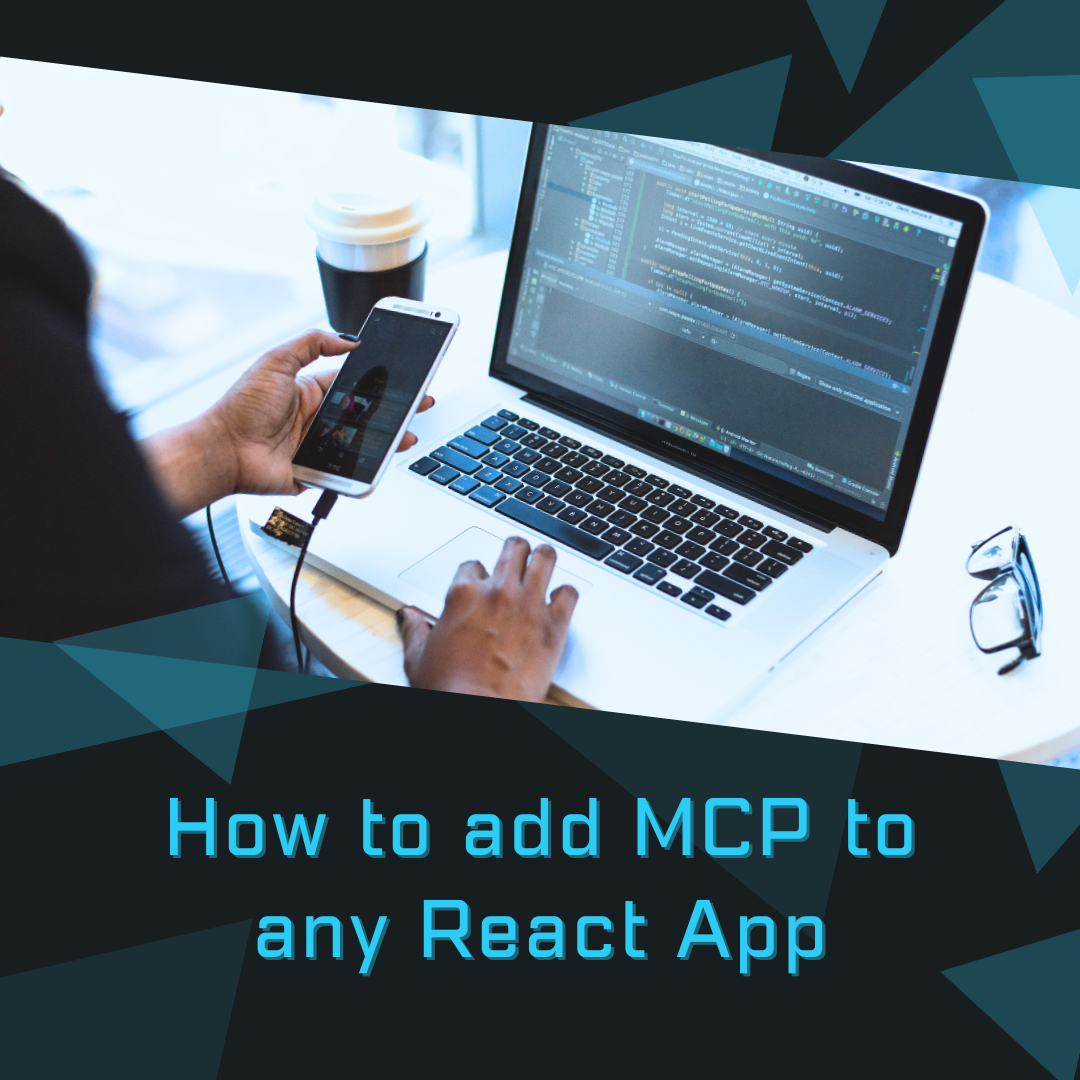
Adding Managed Copilot Protocol (MCP) to a React application enables seamless integration with AI-powered tools and services. This guide walks you through implementing MCP in any React or Next.js app using CopilotKit.
Initial Setup and Project Configuration
If you're starting fresh, create a new Next.js application:
npx create-next-app@latest
For existing projects, initialize MCP support via the CopilotKit CLI:
npx copilotkit@latest init -m MCP
This command:
- Installs required dependencies:
@copilotkit/react-core
and@copilotkit/react-ui
- Configures MCP server connections
- Sets up UI components from the shadcn/ui library
- Handles React 19 compatibility with
--legacy-peer-deps
Installing Core Packages
Install the essential CopilotKit packages:
npm install @copilotkit/react-core @copilotkit/react-ui
@copilotkit/react-core
: Manages MCP server connections and AI context@copilotkit/react-ui
: Provides pre-built chat interface components
Wrapping the Application
Wrap your root layout or main app component with the CopilotKit
provider:
import { CopilotKit } from '@copilotkit/react-core';
export default function RootLayout({ children }) {
return (
<CopilotKit>
{children}
</CopilotKit>
);
}
Don't forget to obtain your API key from Copilot Cloud.
Managing MCP Server Connections
Create a new component McpServerManager.tsx
to manage MCP endpoints dynamically:
'use client';
import { useCopilotChat } from '@copilotkit/react-core';
import { useEffect } from 'react';
export default function McpServerManager() {
const { setMcpServers } = useCopilotChat();
useEffect(() => {
setMcpServers([
{
endpoint: 'https://mcp.composio.dev/gmail',
},
]);
}, []);
return null;
}
Key features:
- Automatically connects to one or more MCP endpoints
- Supports Server-Sent Events (SSE) for real-time communication
- Allows multi-service integration
Tool Call Visualization (Optional Debugging UI)
1. ToolRenderer.tsx
Monitor all tool calls triggered by MCP:
import { useCopilotAction } from '@copilotkit/react-core';
export function ToolRenderer() {
useCopilotAction({
name: '*',
render: ({ status, name, args }) => (
<McpToolCall status={status} name={name} args={args} />
),
});
return null;
}
2. McpToolCall.tsx
Create a collapsible component to visualize each tool call:
export default function McpToolCall({ status, name, args }) {
return (
<div className="border p-2 rounded mb-2">
<strong>{name}</strong>
<div>Status: {status}</div>
<pre>{JSON.stringify(args, null, 2)}</pre>
</div>
);
}
Benefits:
- Real-time feedback on tool execution
- Helpful during development and debugging
- Parses and displays arguments and status
Production Deployment Checklist
1. Environment Variables
COPILOT_CLOUD_PUBLIC_KEY=your_production_key
MCP_ENDPOINTS=https://mcp.yourdomain.com
2. Build Optimization
npm run build
3. Supported Deployment Targets
- Vercel
- Netlify
- AWS Amplify
- Docker Containers
Advanced Configuration
Custom MCP Server Example
setMcpServers([
{
endpoint: 'https://custom.mcp.example.com',
auth: {
type: 'bearer',
token: 'your_auth_token',
},
},
]);
Error Handling
try {
await copilotChat.sendMessage(userInput);
} catch (error) {
console.error('MCP Communication Error:', error);
// Optional: retry or user-facing error message
}
Performance Tips
- Use request caching for repetitive queries
- Delegate heavy processing to Web Workers
- Implement connection pooling in high-traffic scenarios
Security Best Practices
- Always use HTTPS for all endpoints
- Rotate API keys at least quarterly
- Set up rate limiting to avoid abuse
- Use JWT authentication for sensitive actions
- Audit your MCP server configurations regularly
Key Benefits of MCP Integration
- Natural language-powered task execution
- AI-driven automation and smart assistant workflows
- Context-aware service orchestration
- Seamless integration with third-party APIs and backends
Maintenance and Best Practices
- Subscribe to CopilotKit release notes
- Schedule package updates at least once per quarter
- Join the CopilotKit community
- Leverage built-in analytics on Copilot Cloud
Conclusion
Integrating MCP with your React application unlocks powerful AI capabilities that enhance user interaction, automate workflows, and support multi-service orchestration with minimal setup.
With modular architecture and out-of-the-box UI components, CopilotKit enables developers to focus on delivering intelligent user experiences without getting bogged down in backend complexity.
Whether you're building a personal assistant, automating tasks across platforms, or adding natural language support, MCP offers a scalable and secure foundation for AI-powered development.