How to Create Reusable React Components
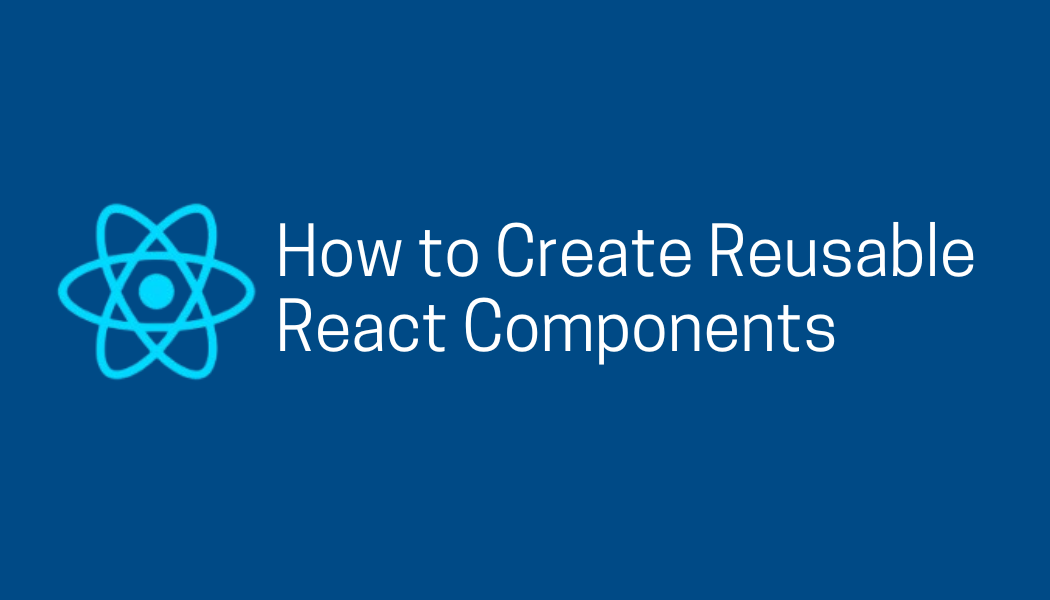
Reusable React components are foundational to building scalable, maintainable, and efficient user interfaces. By abstracting common UI elements and behaviors into self-contained components, developers can reduce code duplication, improve consistency, and accelerate development cycles.
This guide explores the principles, patterns, and practical steps for creating reusable React components—from basic concepts to advanced strategies.
What Is Reusable React Components?
Reusable React components are modular, independent blocks of code that render UI consistently based on the data passed via props. These components are generic, focus on presentation, and avoid side effects or business logic.
For example, instead of creating separate buttons for every use case, you can create a single Button
component that accepts props like color, label, and click handler.
Why Reusability Matters
- Consistency: A unified design language across the app.
- Maintainability: Centralized updates reduce bugs and effort.
- Productivity: Focus on new features, not redundant code.
- Scalability: Easier to expand with modular building blocks.
Principles of Reusable Component Design
1. Single Responsibility Principle (SRP)
Each component should serve one purpose, making it easier to reuse, test, and debug.
2. Avoid Side Effects
Reusable components should avoid fetching data or triggering timers. Let parent components handle side effects and pass data via props.
3. Generic and Configurable
Use props to make components adaptable rather than hardcoding values.
4. Predictable Rendering
Given the same inputs, the component should consistently produce the same output.
Common Examples of Reusable Components
- Buttons: Configurable styles, sizes, and click handlers.
- Inputs: Generic fields for various data types.
- Modals: Flexible overlays for notifications or forms.
- Tables: Data-driven layouts with customizable headers/rows.
- Navbars & Footers: Consistent layout components.
- Cards: Blocks for displaying structured content.
Building a Simple Reusable Component
import React from "react";
const Button = ({ color = "blue", label, onClick }) => (
<button style={{ backgroundColor: color }} onClick={onClick}>
{label}
</button>
);
export default Button;
Usage:
<Button color="green" label="Submit" onClick={handleSubmit} />
Best Practices for Creating Reusable Components
1. Use Props for Customization
Let users customize behavior and appearance without changing internal code.
2. Children Prop for Flexible Content
const Card = ({ children }) => (
<div className="card">
{children}
</div>
);
3. Composition Over Inheritance
Compose smaller components together for better flexibility.
4. Minimal Internal State
Avoid managing internal state unless necessary. Prefer lifting state up and passing callbacks.
5. Avoid Direct Side Effects
No API calls or subscriptions inside reusable components.
6. Style Encapsulation
Use CSS Modules, styled components, or inline styles to prevent style clashes.
Design Patterns for Reusable Components
Pattern | Description | Use Case |
---|---|---|
Container & Presentation | Separate logic from UI | Data fetching component |
Higher-Order Components | Enhance functionality by wrapping components | Authentication wrappers |
Compound Components | Related components share logic via context | Tabs, Accordions |
Provider Pattern | Share state/data using React Context | Theme or Auth Providers |
State Reducer | Control internal state from the outside | Customizable input logic |
Component Composition | Build UIs from smaller reusable parts | Layouts, Cards |
Advanced Techniques
1. Compound Components Pattern
const Tabs = ({ children }) => {
const [activeIndex, setActiveIndex] = React.useState(0);
return (
<div>
{React.Children.map(children, (child, index) =>
React.cloneElement(child, {
isActive: index === activeIndex,
onClick: () => setActiveIndex(index),
})
)}
</div>
);
};
const Tab = ({ isActive, onClick, children }) => (
<button
onClick={onClick}
style={{ fontWeight: isActive ? "bold" : "normal" }}
>
{children}
</button>
);
Usage:
<Tabs>
<Tab>Tab 1</Tab>
<Tab>Tab 2</Tab>
</Tabs>
2. Higher-Order Components (HOC)
function withLogger(WrappedComponent) {
return function (props) {
console.log("Rendering", WrappedComponent.name);
return <WrappedComponent {...props} />;
};
}
Usage:
const LoggedButton = withLogger(Button);
3. Render Props
const List = ({ items, renderItem }) => (
<ul>
{items.map(item => (
<li key={item.id}>{renderItem(item)}</li>
))}
</ul>
);
Usage:
<List items={data} renderItem={item => <span>{item.name}</span>} />
Organizing and Sharing Reusable Components
1. Component Libraries
Centralize common components into a folder or separate package for scalability.
2. Documentation
Use tools like Storybook to document props, states, and behavior.
3. Testing
Write unit and integration tests for behavior, visuals, and accessibility.
Common Pitfalls and How to Avoid Them
- Over-abstracting: Don't make components too generic; tailor them to practical use cases.
- Tight Coupling: Avoid mixing business logic with UI logic.
- Ignoring Accessibility: Use semantic HTML, ARIA roles, and support keyboard navigation.
- Performance Issues: Optimize list rendering and memorize where needed.
Real-World Example: Reusable Input Component
import React from "react";
function Input({ type = "text", placeholder, value, onChange }) {
return (
<input
type={type}
placeholder={placeholder}
value={value}
onChange={onChange}
/>
);
}
export default Input;
Usage:
<Input
placeholder="Email"
value={email}
onChange={e => setEmail(e.target.value)}
/>
Making Complex Components Reusable: Navbar Example
const Navbar = ({ logo, links, user }) => (
<nav className="navbar">
<div className="logo">{logo}</div>
<ul>
{links.map(link => (
<li key={link.href}>
<a href={link.href}>{link.label}</a>
</li>
))}
</ul>
<div>{user ? <UserProfile /> : <LoginButton />}</div>
</nav>
);
This flexible layout supports branding, dynamic links, and conditional rendering for logged-in users.
Testing Reusable Components
- Unit Tests: Validate rendering and prop handling.
- Snapshot Tests: Detect unexpected UI changes.
- Accessibility Tests: Ensure usability for all users using tools like axe.
Sharing Components Across Projects
- NPM Packages: Publish reusable components for use across apps.
- Bit.dev: Share components without creating full packages.
- Monorepos: Manage shared components in a unified repository.
Conclusion
Reusable React components form the backbone of scalable front-end development. By adhering to best practices—like SRP, prop-driven design, and leveraging advanced patterns like HOCs and compound components—you can create a maintainable, flexible codebase.
These components not only accelerate development but also enforce design consistency and improve code quality. As your application grows, your reusable component library becomes a crucial asset for speed, innovation, and reliability.
"Reusable React components are those components that are pure and predictable. These components are free from complex business logic and are generic in nature. Based on the data that is passed to these components, they should render consistent user interfaces."