Install & Run Stable Code 3B on Windows
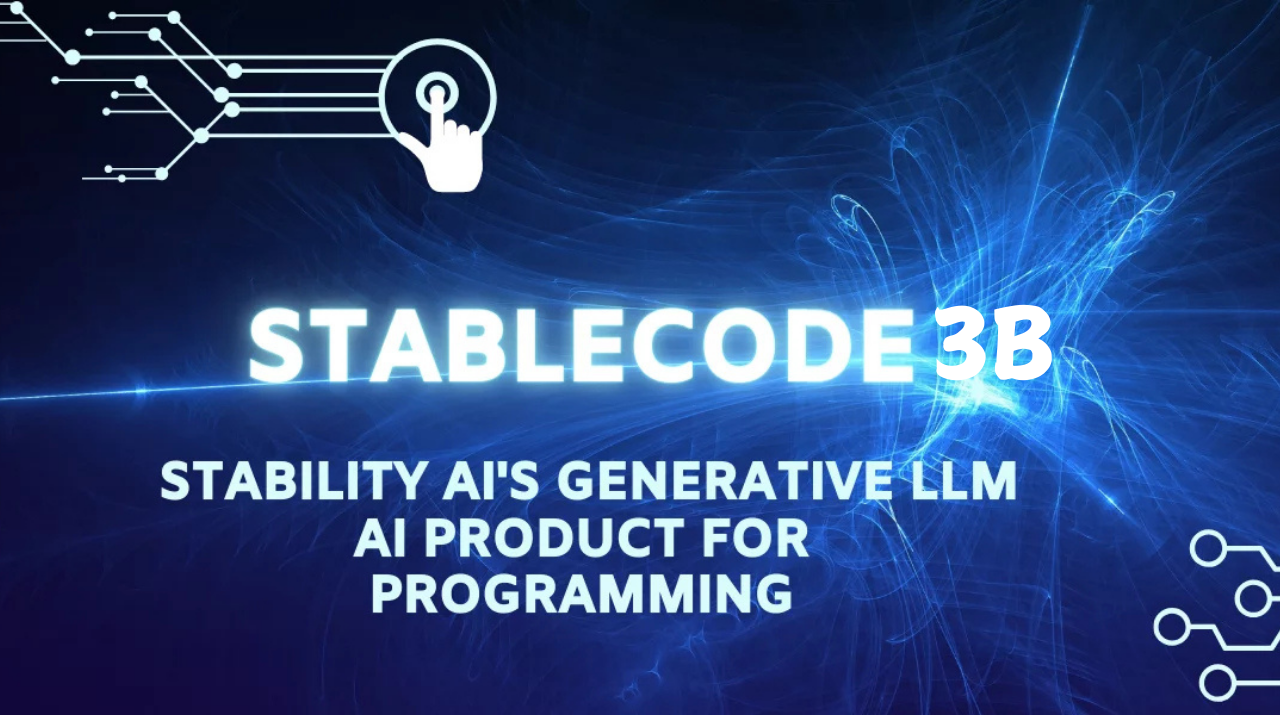
The installation and execution of Stable Code 3B on a Windows system necessitate a systematic approach, encompassing environment configuration, dependency management, and model execution.
Overview of Stable Code 3B
Stable Code 3B, an advanced autoregressive transformer model engineered by Stability AI, is explicitly optimized for code generation and completion across 18 programming languages, including Python, Java, and C++.
It consists of 2.7 billion parameters and employs a decoder-only architecture akin to Meta’s LLaMA, facilitating sophisticated contextual inference.
Salient Features
- Resource Efficiency: Engineered for execution on conventional computing hardware without dedicated GPUs.
- Computational Superiority: Demonstrates competitive performance relative to larger-scale models in domain-specific code generation tasks.
- Extended Contextual Understanding: Processes input sequences up to 100,000 tokens, enabling superior comprehension of extensive codebases.
Preinstallation Considerations
Prior to initializing the installation, ensure the system satisfies the following conditions:
- Operating System: Windows 10 or later.
- Python Runtime: Version 3.8 or higher.
- Package Management:
pip
installed and up to date. - Version Control (Optional): Git installed for repository management.
Procedural Installation Instructions
Step 1: Python Installation
- Retrieve the latest Python release from the official Python repository.
- Execute the installation process, ensuring the "Add Python to PATH" option is selected.
Complete the setup and verify the installation using:
python --version
Step 2: Dependency Acquisition
Utilize the following command to install requisite libraries:
pip install torch transformers huggingface-hub
Step 3: Model Retrieval from Hugging Face
Authenticate with Hugging Face:
huggingface-cli login
Proceed with model acquisition:
mkdir stable-code-3b
huggingface-cli download stabilityai/stable-code-3b --local-dir stable-code-3b --local-dir-use-symlinks False
Step 4: Environment Configuration
Deploy a development environment by utilizing an IDE such as VSCode or PyCharm, ensuring seamless script execution.
Step 5: Model Execution
Create run_stable_code.py
and incorporate the following implementation:
import torch
from transformers import AutoModelForCausalLM, AutoTokenizer
# Initialize tokenizer and model
tokenizer = AutoTokenizer.from_pretrained("stabilityai/stable-code-3b", trust_remote_code=True)
model = AutoModelForCausalLM.from_pretrained("stabilityai/stable-code-3b", trust_remote_code=True)
# Deploy model to GPU if available
if torch.cuda.is_available():
model.cuda()
# Define input sequence
input_text = "def hello_world():\n print('Hello, world!')"
inputs = tokenizer(input_text, return_tensors="pt").to(model.device)
# Generate response
tokens = model.generate(inputs['input_ids'], max_new_tokens=48, temperature=0.2)
output = tokenizer.decode(tokens[0], skip_special_tokens=True)
print(output)
Step 6: Execution of the Model
Navigate to the script’s directory and execute:
python run_stable_code.py
This will yield code generation output based on the provided input prompt.
Advanced Practical Implementations
Example 1: Flask-Based RESTful API
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/greet', methods=['GET'])
def greet():
return jsonify({"message": "Hello, World!"})
if __name__ == '__main__':
app.run(debug=True)
Example 2: Dynamic SQL Query Generation
input_text = "Construct an SQL query to retrieve all users aged over 30."
inputs = tokenizer(input_text, return_tensors="pt").to(model.device)
tokens = model.generate(inputs['input_ids'], max_new_tokens=100)
sql_query = tokenizer.decode(tokens[0], skip_special_tokens=True)
print(sql_query)
Example 3: Data Processing with Pandas
import pandas as pd
data = {"Name": ["Alice", "Bob", "Charlie"], "Age": [25, 30, 35]}
df = pd.DataFrame(data)
print(df[df["Age"] > 28])
Troubleshooting and Optimization Strategies
- CUDA Compatibility Issues: If GPU acceleration is unavailable, verify CUDA installation and driver compatibility.
- Model Download Failures: Reauthenticate via
huggingface-cli
or confirm network stability.
Dependency Conflicts: Ensure pip
is updated by executing:
pip install --upgrade pip
Conclusion
The model's advanced code-generation capabilities facilitate enhanced productivity and seamless integration into diverse software development workflows. Further exploration of its potential can yield significant advancements in automated programming assistance and large-scale code analysis.