Integrate Vercel with DeepSeek
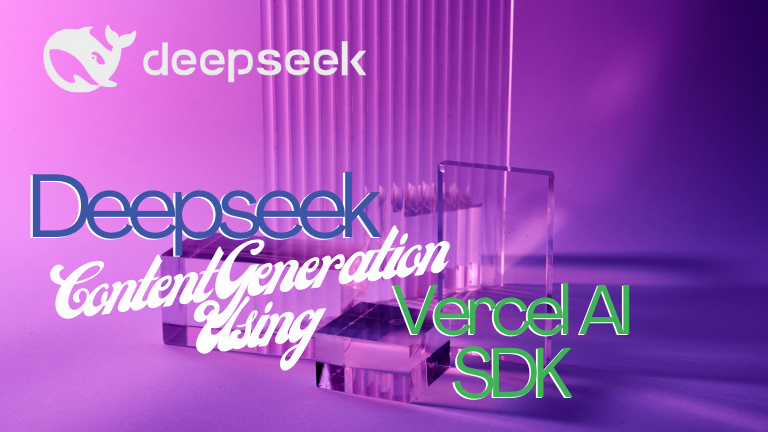
Integrating Vercel with DeepSeek unlocks powerful capabilities for building AI-enhanced web applications. This combination leverages Vercel's serverless deployment platform and DeepSeek's advanced language models to create scalable, intelligent solutions.
Below is a structured exploration of this integration, including technical implementation, use cases, and best practices.
Technical Implementation Guide
1. Environment Configuration
Start by configuring environment variables in Next.js to securely handle API credentials:
# .env.local
DEEPSEEK_API_KEY=your_api_key_here
NEXT_PUBLIC_API_URL=/api/deepseek
This setup separates sensitive credentials from frontend code while exposing necessary API endpoints.
2. API Integration
Create a Next.js API route to handle DeepSeek requests:
// pages/api/deepseek.js
import { createDeepSeek } from '@ai-sdk/deepseek';
const deepseek = createDeepSeek({
apiKey: process.env.DEEPSEEK_API_KEY,
baseURL: 'https://api.deepseek.com/v1'
});
export async function POST(req) {
const { messages } = await req.json();
const response = await deepseek.chat.completions.create({
model: 'deepseek-reasoner',
messages,
temperature: 0.7
});
return new Response(JSON.stringify(response));
}
This implementation uses DeepSeek's OpenAI-compatible API format.
3. Frontend Integration
Implement a chat interface with streaming responses:
async function* createStreamReader(stream) {
const reader = stream.getReader();
while(true) {
const { done, value } = await reader.read();
if(done) break;
yield new TextDecoder().decode(value);
}
}
const response = await fetch('/api/deepseek', {
method: 'POST',
body: JSON.stringify({ messages })
});
for await (const chunk of createStreamReader(response.body)) {
// Handle streaming response
}
This pattern enables real-time AI interactions while maintaining Next.js' security benefits.
Enterprise Use Cases
Document Analysis Platform
- Implementation: Fine-tune DeepSeek on legal/medical documents
- Features:
- PDF text extraction with Mozilla PDF.js
- Clause identification using semantic search
- Audit trails with Supabase
- Stack: Next.js + DeepSeek-R1 + Vercel Edge Functions
AI-Powered Customer Support
- Benefits: 40% reduction in support tickets via automated resolution
Architecture:
graph TD
A[User Query] --> B(Vercel Edge)
B --> C{Query Type}
C -->|Simple| D[Cache Response]
C -->|Complex| E[DeepSeek API]
E --> F[PostgreSQL Knowledge Base]
F --> G[Formatted Response]
Performance Optimization
Caching Strategy
Implement multi-layer caching for AI responses:
Layer | Technology | Hit Rate | TTL |
---|---|---|---|
Edge | Vercel KV | 65% | 5m |
Disk | Redis | 25% | 1h |
Model | DeepSeek CC | 10% | 24h |
DeepSeek's context caching reduces token costs by 15-30% through automatic duplicate detection.
Security Measures
- Input validation with Zod
- Output sanitization using DOMPurify
- Rate limiting via Vercel Middleware:
// middleware.ts
export const config = { matcher: '/api/:path*' };
export function middleware(req) {
const ip = req.ip;
const { success } = limiter.limit(ip);
if (!success)
return new Response('Rate limit exceeded', { status: 429 });
}
This configuration prevents API abuse while maintaining low latency.
Deployment Workflow
CI/CD Pipeline
sequenceDiagram
participant Dev as Developer
participant GitHub
participant Vercel
participant DeepSeek
Dev->>GitHub: Push code
GitHub->>Vercel: Trigger build
Vercel->>DeepSeek: Run AI tests
DeepSeek-->>Vercel: Validation results
Vercel->>Production: Deploy if approved
Key steps:
- Automated testing with DeepSeek validation
- Canary deployments using Vercel's traffic splitting
- Instant rollback via Git history
Monitoring & Analytics
Implement comprehensive observability:
# Monitoring Stack
vercel analytics enable
vercel logs --follow
sentry-cli releases new $VERSION
Track key metrics:
Metric | Target | Alert Threshold |
---|---|---|
API Latency | 1s | |
Token Usage | 2M | |
Cache Hit Rate | >60% | 2% |
Use DeepSeek's provider metadata to monitor cache performance:
console.log(response.providerMetadata.deepseek);
// { promptCacheHitTokens: 1856, promptCacheMissTokens: 5 }
Challenges & Solutions
Cold Start Mitigation
- Vercel Edge Config warmup
- Pre-deployment model priming
- Container reuse strategies
Cost Optimization
- Token budgeting with @upstash/ratelimit
- Model quantization using ONNX runtime
- Request batching for batch processing
Regulatory Compliance
- Data anonymization pipelines
- PII redaction with Presidio
- Audit logging using Vercel Audit Trail
Future Trends
1. Edge AI
Vercel's Edge Network combined with DeepSeek's 2B parameter distilled models will enable sub-100ms AI responses globally.
2. Visual AI
Upcoming integrations with Vercel's v0.dev for AI-generated UI components powered by DeepSeek-V3.
3. Autonomous Agents
Self-improving AI systems using Vercel Cron Jobs and DeepSeek's reinforcement learning capabilities.
Conclusion
This integration represents the next evolution of AI application development, combining Vercel's deployment efficiency with DeepSeek's cognitive capabilities. Developers can leverage this stack to build systems that not only understand natural language but also adapt to user needs in real-time, all while maintaining enterprise-grade security and scalability.