JavaScript Date Object is Replaced: How to use it!
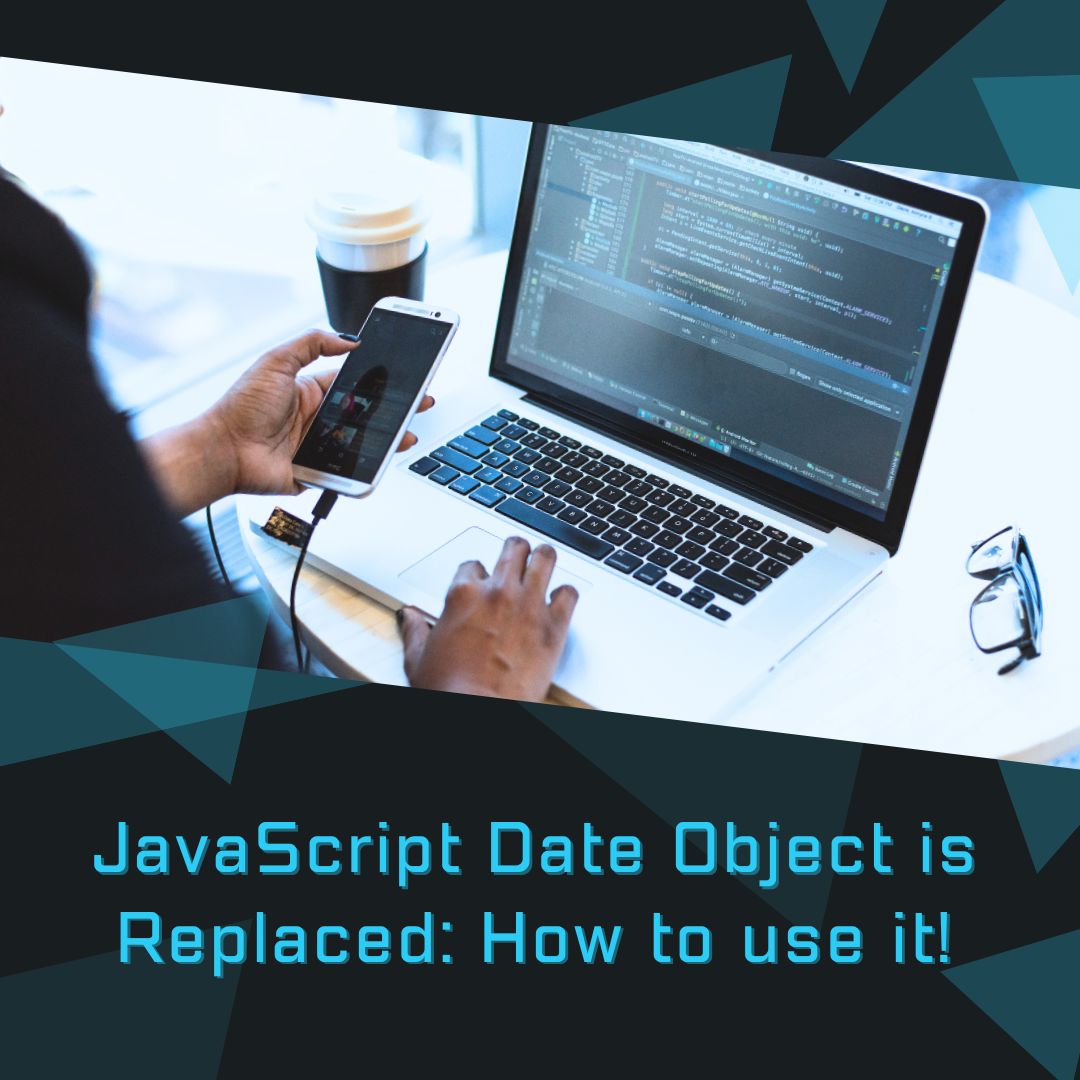
The JavaScript Date
object has long powered everything from clocks to calendars, but it's also been a major source of confusion and bugs. Now, in 2025, JavaScript is undergoing a significant shift: the Date
object is being replaced by the modern, robust Temporal
API—a long-awaited upgrade for date and time handling.
This guide breaks down why Date
is being replaced, the problems it posed, and how to effectively use the Temporal
API in your JavaScript applications.
The Legacy of JavaScript’s Date Object
Origins and Limitations
Introduced in 1995 and modeled after Java’s early date-handling classes, JavaScript’s Date
object was never ideal. Java moved on quickly; JavaScript didn’t.
Key problems with the Date object:
- Limited Timezone Support: Only supports UTC and local time.
- Inconsistent Parsing: Varies by browser and locale.
- Mutable Objects: Changes to a
Date
can lead to hard-to-trace bugs. - Daylight Saving Time Issues: Transitions are hard to calculate correctly.
- No Duration or Interval Support: Requires third-party libraries.
Developers often turned to libraries like Moment.js, date-fns, and Luxon to patch these shortcomings.
Introducing the Temporal API
What Is Temporal?
The Temporal
API is a modern JavaScript API that solves the issues inherent in the Date
object. It’s built into the language and provides precise, immutable, and timezone-aware tools for handling date and time.
Highlights of Temporal:
- Immutable Objects
- Native Timezone and Calendar Support
- Reliable ISO Parsing
- Extensive Utility Methods
- Clear Concept Separation (dates, times, instants, durations)
“Temporal is designed as a full replacement for the Date object, making date and time management reliable and predictable.” — MDN Web Docs
Comparing Date and Temporal
Feature | Date Object | Temporal API |
---|---|---|
Timezone support | UTC and local only | Full IANA time zone support |
Mutability | Mutable | Immutable |
Parsing | Inconsistent | Reliable ISO 8601 and more |
DST handling | Error-prone | Built-in support |
Duration/intervals | Not supported | Fully supported |
Formatting | Limited | Locale-aware via Intl |
API depth | Minimal | Comprehensive |
Getting Started with Temporal
Key Classes in Temporal
Temporal.PlainDate
: Date onlyTemporal.PlainTime
: Time onlyTemporal.PlainDateTime
: Combined date and timeTemporal.ZonedDateTime
: Date/time with time zoneTemporal.Instant
: Exact moment in timeTemporal.Duration
: Amount of timeTemporal.Calendar
: Calendar systemsTemporal.TimeZone
: Time zone identifiers
Creating Temporal Objects
const date = Temporal.PlainDate.from('2025-05-03');
const time = Temporal.PlainTime.from('14:30:00');
const dateTime = Temporal.PlainDateTime.from('2025-05-03T14:30:00');
const zoned = Temporal.ZonedDateTime.from({
year: 2025, month: 5, day: 3, hour: 14, minute: 30,
timeZone: 'Asia/Kolkata'
});
const now = Temporal.Now.instant();
Working with Time Zones and Calendars
Time Zone Example
const nyTime = Temporal.ZonedDateTime.from({
year: 2025, month: 5, day: 3, hour: 10, minute: 0,
timeZone: 'America/New_York'
});
Calendar Example
const japaneseDate = Temporal.PlainDate.from({
year: 2025, month: 5, day: 3, calendar: 'japanese'
});
Manipulating Dates and Times
Adding and Subtracting
const date = Temporal.PlainDate.from('2025-05-03');
const nextWeek = date.add({ days: 7 });
Comparisons
const d1 = Temporal.PlainDate.from('2025-05-03');
const d2 = Temporal.PlainDate.from('2025-05-10');
console.log(d1.equals(d2)); // false
console.log(d1.until(d2).days); // 7
Durations
const duration = Temporal.Duration.from({ days: 3, hours: 5 });
Formatting and Parsing
Formatting with Locale
const date = Temporal.PlainDate.from('2025-05-03');
const formatted = date.toLocaleString('en-US', { dateStyle: 'full' });
// "Saturday, May 3, 2025"
Parsing
const date = Temporal.PlainDate.from('2025-05-03');
Converting Between Temporal Types
const instant = Temporal.Now.instant();
const zoned = instant.toZonedDateTimeISO('Asia/Kolkata');
Migrating from Date to Temporal
Convert Date
to Temporal
const date = new Date();
const instant = Temporal.Instant.fromEpochMilliseconds(date.getTime());
Convert Temporal
to Date
const instant = Temporal.Now.instant();
const jsDate = new Date(instant.epochMilliseconds);
Practical Examples
Scheduling in Different Time Zones
const meetingUTC = Temporal.ZonedDateTime.from({
year: 2025, month: 5, day: 3, hour: 9, minute: 0, timeZone: 'UTC'
});
const localMeeting = meetingUTC.withTimeZone('Asia/Kolkata');
Calculating Durations
const start = Temporal.PlainDateTime.from('2025-05-03T09:00');
const end = Temporal.PlainDateTime.from('2025-05-03T17:30');
const duration = start.until(end);
// "PT8H30M"
Daylight Saving Time Example
const beforeDST = Temporal.ZonedDateTime.from({
year: 2025, month: 3, day: 9, hour: 1, timeZone: 'America/New_York'
});
const afterDST = beforeDST.add({ hours: 2 });
Internationalization Support
const date = Temporal.PlainDate.from('2025-05-03');
const formatter = new Intl.DateTimeFormat('fr-FR', { dateStyle: 'full' });
console.log(formatter.format(date)); // "samedi 3 mai 2025"
Best Practices and Common Pitfalls
- Temporal objects are immutable.
- Always use
ZonedDateTime
when dealing with time zones. - Prefer ISO 8601 format for parsing.
- Use
Temporal.Instant
when converting from legacyDate
.
Browser Support and Polyfills
As of 2025, Temporal is available in experimental browser builds, but not universally supported. Use the proposal-temporal polyfill for broader compatibility in production environments.
Libraries and Compatibility
While libraries like Moment.js, date-fns, and Luxon remain relevant, most are transitioning to leverage Temporal
under the hood.
Conclusion
The new Temporal API transforms JavaScript’s handling of dates and times. It eliminates the legacy issues of the Date
object and introduces a precise, powerful, and modern standard for developers.