Project Ideas in ASP.NET C#
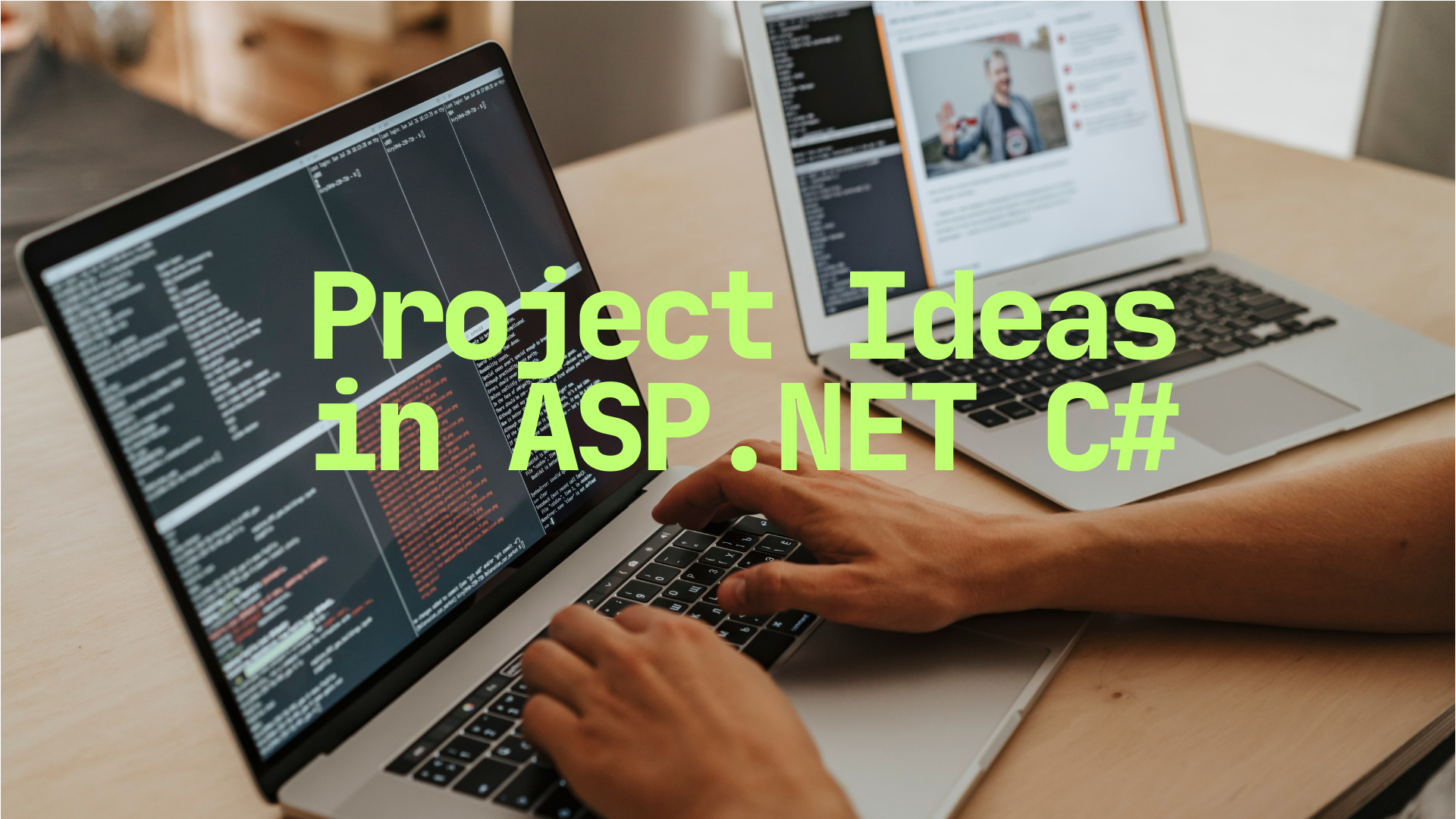
The ASP.NET framework, in conjunction with C#, serves as an integral toolset for the development of enterprise-grade web applications. As a mature and feature-rich framework developed by Microsoft, ASP.NET facilitates the creation of highly scalable, maintainable, and secure applications.
This article presents an array of project ideas categorized by complexity, offering insights into their architectural considerations, implementation methodologies, and potential challenges.
Theoretical Underpinnings of ASP.NET and C#
A comprehensive understanding of ASP.NET and C# is essential before embarking on project development.
- ASP.NET: A robust web development framework that supports Model-View-Controller (MVC) architecture, dependency injection, and middleware configuration, among other advanced paradigms.
- C#: A statically-typed, object-oriented programming language optimized for interoperability within the .NET ecosystem, featuring attributes such as asynchronous programming via
async/await
, LINQ for data querying, and an extensive standard library for enterprise application development.
Foundational Project Implementations
These beginner-level projects establish core competencies in CRUD operations, authentication mechanisms, and relational database interactions.
1. To-Do List Application
- Objective: Implement a structured approach to task management.
- Core Features: User authentication, CRUD operations, and persistent storage.
- Technologies: ASP.NET Core, C#, Entity Framework Core, SQL Server.
Code Illustration:
public class TaskItem
{
public int Id { get; set; }
public string Description { get; set; }
public bool IsCompleted { get; set; }
}
2. Personal Finance Tracker
- Objective: Develop an application for expense tracking and financial reporting.
- Core Features: User authentication, transaction categorization, financial visualization.
- Technologies: ASP.NET Core, C#, Chart.js for data visualization.
Code Illustration:
public class Transaction
{
public int Id { get; set; }
public string Category { get; set; }
public decimal Amount { get; set; }
public DateTime Date { get; set; }
}
Intermediate-Level Architectural Constructs
These projects introduce service-oriented architecture (SOA), payment gateway integration, and API consumption.
1. E-commerce Web Application
- Objective: Construct a secure online retail system.
- Core Features: Shopping cart, order management, payment processing.
- Technologies: ASP.NET Core MVC, C#, Stripe API.
Code Illustration:
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
2. Online Learning Management System (LMS)
- Objective: Develop a platform for online course management and student enrollment.
- Core Features: Course creation, student authentication, progress tracking.
- Technologies: ASP.NET Core MVC, C#, SQL Server.
Code Illustration:
public class Course
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
}
3. Healthcare Appointment Booking System
- Objective: Facilitate online scheduling of medical consultations.
- Core Features: Patient registration, doctor availability tracking, appointment notifications.
- Technologies: ASP.NET Core MVC, C#, Entity Framework Core.
Code Illustration:
public class Appointment
{
public int Id { get; set; }
public int PatientId { get; set; }
public int DoctorId { get; set; }
public DateTime AppointmentDate { get; set; }
}
Advanced-Level Project Implementations
For seasoned developers, the following projects emphasize complex domains such as IoT, blockchain, and AI-driven applications.
1. IoT-Based Home Automation System
- Objective: Enable real-time device control and monitoring.
- Core Features: MQTT-based messaging, WebSockets, data analytics.
- Technologies: ASP.NET Core, C#, MQTT, Raspberry Pi.
Code Illustration:
public class IoTDevice
{
public int Id { get; set; }
public string DeviceName { get; set; }
public bool Status { get; set; }
}
2. Blockchain-Based Supply Chain Management System
- Objective: Implement a decentralized tracking system for supply chains.
- Core Features: Smart contracts, real-time tracking, authentication mechanisms.
- Technologies: ASP.NET Core, C#, Ethereum Blockchain.
Code Illustration:
public class SupplyChainItem
{
public int Id { get; set; }
public string ProductName { get; set; }
public string Status { get; set; }
}
3. AI-powered chatbot for Customer Support
- Objective: Develop an AI-based chatbot to assist users in real time.
- Core Features: Natural Language Processing (NLP), automated responses, sentiment analysis.
- Technologies: ASP.NET Core, C#, OpenAI API.
Code Illustration:
public class ChatMessage
{
public int Id { get; set; }
public string UserMessage { get; set; }
public string BotResponse { get; set; }
}
Conclusion
Engaging in ASP.NET C# projects at varying levels of complexity enhances proficiency in enterprise software development. Developers can cultivate expertise in application architecture, security paradigms, and emerging technological integrations by systematically exploring these projects.
A continuous focus on learning and adapting to novel programming paradigms ensures the development of sophisticated and scalable software solutions.