Top 10 Best React Design Patterns
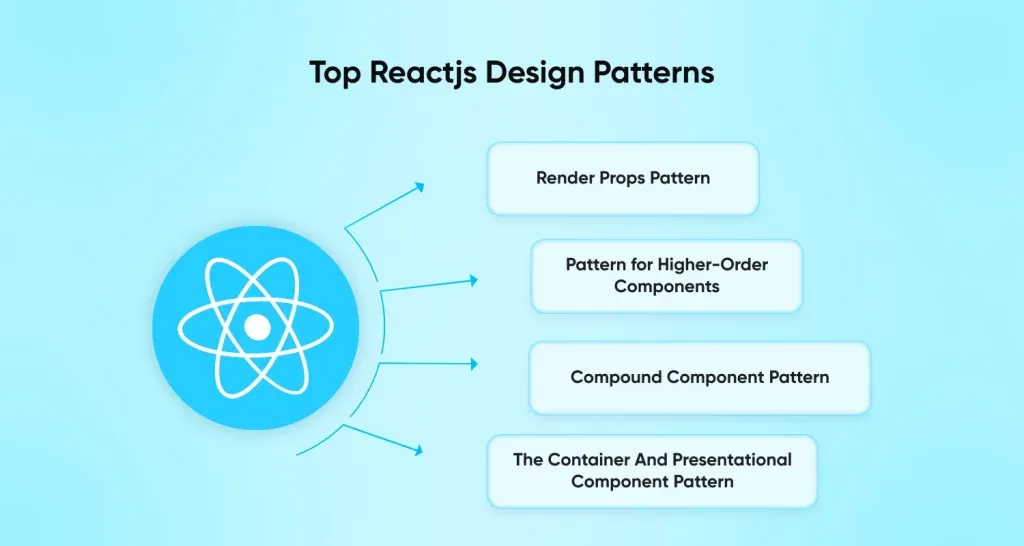
React has matured into a powerful library for building user interfaces, and with its evolution comes a set of design patterns that help developers write scalable, maintainable, and efficient applications. Whether you're creating a complex enterprise dashboard or a sleek e-commerce site, leveraging the right design patterns is key to success.
Below is a comprehensive, reader-friendly guide to the Top 10 React Design Patterns in 2025, complete with use cases, benefits, and code examples.
1. Container and Presentational Components
Concept:
Separates data logic from UI rendering by dividing components into:
- Container Components: Manage state, side effects, and business logic.
- Presentational Components: Focus purely on UI and receive data via props.
Benefits:
- Enhances reusability and testability
- Decouples logic from UI
- Simplifies maintenance
Example:
// Container Component
class UserContainer extends React.Component {
state = { user: null };
componentDidMount() {
fetchUser().then(user => this.setState({ user }));
}
render() {
return <UserProfile user={this.state.user} />;
}
}
// Presentational Component
const UserProfile = ({ user }) => (
<div>{user ? user.name : 'Loading...'}</div>
);
Use Case:
Best suited for large-scale apps like dashboards and e-commerce platforms.
2. Higher-Order Components (HOCs)
Concept:
A HOC is a function that takes a component and returns an enhanced component with additional functionality.
Benefits:
- Promotes DRY (Don’t Repeat Yourself) code
- Encourages logic reuse
- Separates concern cleanly
Example:
const withUserData = (Component) => {
return class extends React.Component {
state = { user: null };
componentDidMount() {
fetchUser().then(user => this.setState({ user }));
}
render() {
return <Component user={this.state.user} />;
}
};
};
const UserProfile = ({ user }) => <div>{user ? user.name : 'Loading...'}</div>;
const UserProfileWithUserData = withUserData(UserProfile);
Use Case:
Great for authentication wrappers, feature toggles, and analytics.
3. Render Props
Concept:
Pass a function as a prop to dynamically determine what a component renders.
Benefits:
- Enables logic sharing between components
- Offers UI flexibility
- Avoids inheritance complexities
Example:
class MouseTracker extends React.Component {
state = { x: 0, y: 0 };
handleMouseMove = (e) => {
this.setState({ x: e.clientX, y: e.clientY });
};
render() {
return (
<div onMouseMove={this.handleMouseMove}>
{this.props.render(this.state)}
</div>
);
}
}
// Usage
<MouseTracker render={({ x, y }) => <p>Mouse position: {x}, {y}</p>} />
Use Case:
Perfect for custom logic like mouse tracking, animations, and validation.
4. Compound Components
Concept:
A parent component shares state with its children, allowing a declarative and flexible API.
Benefits:
- Provides intuitive API for complex UIs
- Encourages composition and flexibility
- Simplifies shared state management
Example:
const Dropdown = ({ children }) => {
const [isOpen, setIsOpen] = React.useState(false);
return (
<div>
<button onClick={() => setIsOpen(!isOpen)}>Toggle</button>
{isOpen && <div>{children}</div>}
</div>
);
};
const DropdownItem = ({ children }) => <div>{children}</div>;
// Usage
<Dropdown>
<DropdownItem>Item 1</DropdownItem>
<DropdownItem>Item 2</DropdownItem>
</Dropdown>
Use Case:
Best for tabs, modals, accordions, and dropdowns.
5. Hooks and Custom Hooks
Concept:
Hooks allow stateful logic in functional components. Custom hooks extract reusable logic into functions.
Benefits:
- Enables logic reuse without HOCs
- Reduces boilerplate
- Enhances readability
Example:
const useFetchUser = () => {
const [user, setUser] = React.useState(null);
React.useEffect(() => {
fetchUser().then(setUser);
}, []);
return user;
};
const UserProfile = () => {
const user = useFetchUser();
return <div>{user ? user.name : 'Loading...'}</div>;
};
Use Case:
Ideal for data fetching, form management, and side effects.
6. Provider Pattern (Context API)
Concept:
Uses React Context to share global data without prop drilling.
Benefits:
- Simplifies global state sharing
- Eliminates deeply nested props
- Centralizes configuration and themes
Example:
const ThemeContext = React.createContext();
function App() {
return (
<ThemeContext.Provider value="dark">
<Toolbar />
</ThemeContext.Provider>
);
}
function Toolbar() {
const theme = React.useContext(ThemeContext);
return <div>Theme: {theme}</div>;
}
Use Case:
Useful for themes, authentication, language settings, and more.
7. Controlled Components
Concept:
Form elements controlled by React state, offering tight control and predictability.
Benefits:
- Single source of truth
- Easier validation and testing
- More robust form handling
Example:
function ControlledInput() {
const [value, setValue] = React.useState('');
return (
<input
type="text"
value={value}
onChange={(e) => setValue(e.target.value)}
/>
);
}
Use Case:
Ideal for forms, search inputs, and live validations.
8. State Reducer Pattern
Concept:
Let's users control how state transitions happen within a component via a reducer.
Benefits:
- Full control over internal state logic
- Advanced customization
- Encourages clean separation of concerns
Example:
function useToggle({ initial = false, reducer }) {
const [state, dispatch] = React.useReducer(reducer, { on: initial });
const toggle = () => dispatch({ type: 'toggle' });
return [state.on, toggle];
}
function toggleReducer(state, action) {
switch (action.type) {
case 'toggle':
return { on: !state.on };
default:
return state;
}
}
Use Case:
Best for highly reusable UI components like toggles or accordions.
9. Extensible Styles Pattern
Concept:
Components accept custom styles or class names as props for greater customization.
Benefits:
- Flexible theming support
- Easy overrides for design systems
- Plays well with CSS-in-JS
Example:
function Button({ className = '', style, ...props }) {
return <button className={`btn ${className}`} style={style} {...props} />;
}
Use Case:
Great for component libraries and customizable UI kits.
10. Prop Combination Pattern
Concept:
Combine related props into a single object for cleaner, more scalable code.
Benefits:
- Cleaner API
- Easier prop management
- Scales better with complexity
Example:
function P({ style, children, ...rest }) {
return <p style={style} {...rest}>{children}</p>;
}
function App() {
const paragraphProps = { style: { color: 'red', fontSize: '20px', lineHeight: '22px' } };
return <P {...paragraphProps}>This is a paragraph</P>;
}
Use Case:
Ideal for passing style or config objects in larger apps.
Comparison Table
Pattern | Main Use Case | Key Benefit |
---|---|---|
Container/Presentational | Separation of concerns | Reusability, maintainability |
Higher-Order Components (HOC) | Logic sharing | DRY, code reuse |
Render Props | Dynamic rendering | Flexibility, code sharing |
Compound Components | Complex UIs | Declarative, flexible API |
Hooks & Custom Hooks | State & logic reuse | Clean, modern code |
Provider Pattern (Context) | Global state sharing | No prop drilling |
Controlled Components | Forms/input | Predictable, testable |
State Reducer Pattern | Custom state logic | Advanced customization |
Extensible Styles Pattern | Theming, component libraries | Flexible styling |
Prop Combination Pattern | Prop management | Readable, scalable |
Conclusion
Mastering these React design patterns empowers developers to build robust, scalable, and maintainable applications in 2025 and beyond. Whether you're aiming for better performance, reusability, or developer experience, each pattern plays a crucial role in solving specific challenges.
By strategically incorporating these patterns into your projects, you ensure cleaner codebases, easier collaboration, and a more professional development workflow.