Top 10 HTML Projects
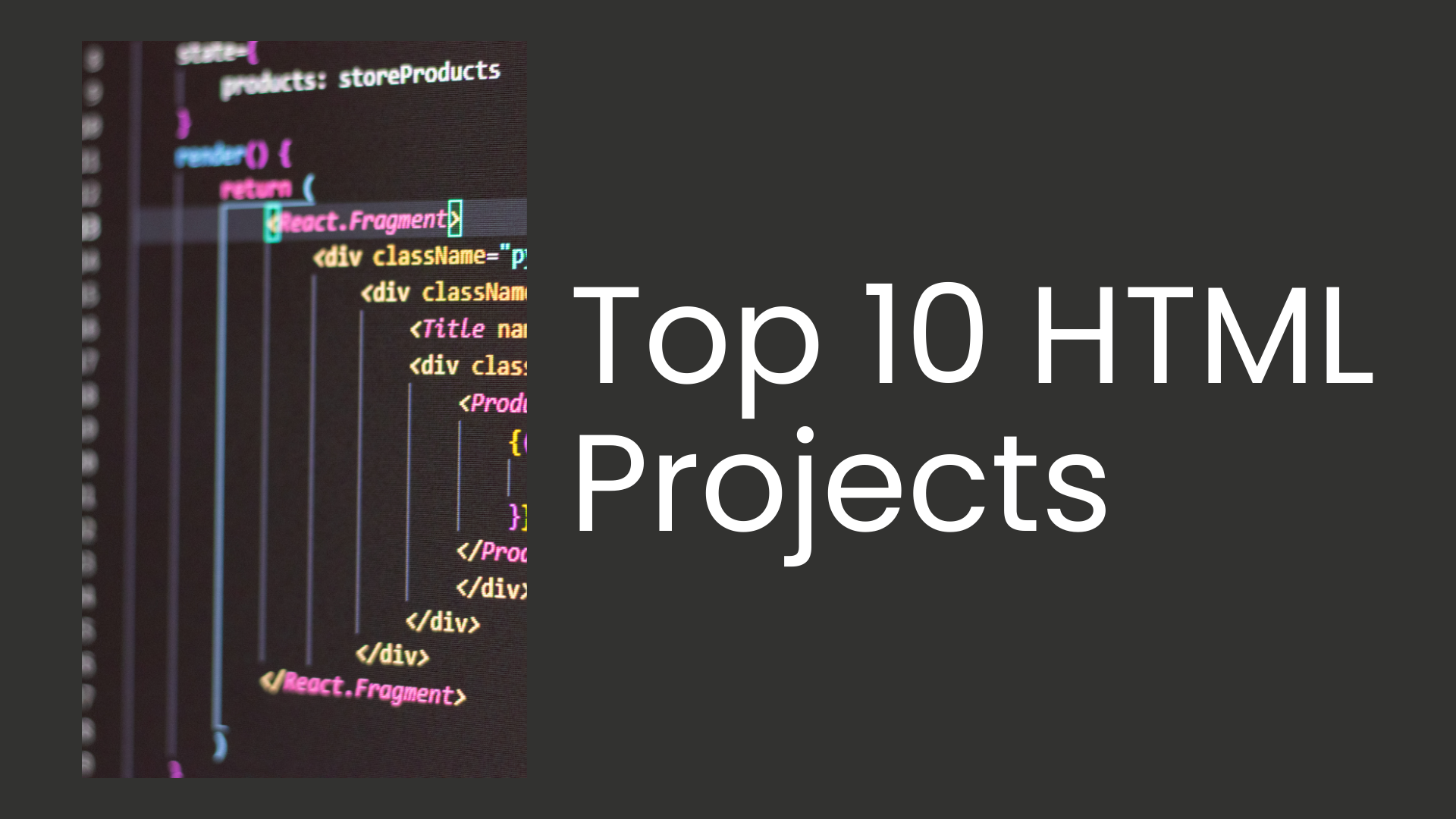
HTML serves as the fundamental framework for web development, and engaging in hands-on projects is an optimal strategy for deepening one's understanding of its core principles.
The following ten HTML projects, ranging from elementary to sophisticated, provide opportunities to refine technical competencies and cultivate a portfolio of professional-grade web applications.
1. Personal Portfolio Website
A personal portfolio website is an essential digital asset that allows professionals to exhibit their expertise, work history, and accomplishments in a structured and aesthetically appealing manner.
Key Features:
- Segmented layout featuring an introduction, competencies, projects, and contact information.
- Integration of multimedia elements such as images, videos, or animations.
- Adaptive design to ensure cross-device accessibility.
Technologies Required:
- HTML for content structure.
- CSS for visual styling and layout organization.
- JavaScript (optional) for enhanced interactivity.
Example Code:
<!DOCTYPE html>
<html>
<head>
<title>Professional Portfolio</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<header>
<h1>Dr. Jane Doe</h1>
<p>Web Development Specialist</p>
</header>
<section id="about">
<h2>About Me</h2>
<p>With a strong background in front-end development, I specialize in crafting immersive digital experiences.</p>
</section>
</body>
</html>
2. Tribute Page
A tribute page is a concise and informative webpage dedicated to an influential individual, integrating textual and graphical elements.
Key Features:
- Prominent header featuring the subject’s name and image.
- Sections delineating biography, contributions, and notable quotes.
- Enhanced styling for an aesthetically coherent presentation.
Example Code:
<!DOCTYPE html>
<html>
<head>
<title>Tribute Page</title>
</head>
<body>
<h1>Tribute to Marie Curie</h1>
<img src="curie.jpg" alt="Marie Curie">
<p>Marie Curie was a pioneering physicist and chemist renowned for her research on radioactivity.</p>
</body>
</html>
3. Landing Page Design
A landing page is a strategically designed single-page site optimized for marketing purposes, aimed at maximizing user engagement and conversions.
Example Code:
<!DOCTYPE html>
<html>
<head>
<title>Innovative Product</title>
</head>
<body>
<header>
<h1>Discover the Future</h1>
<button>Sign Up Now</button>
</header>
</body>
</html>
4. Parallax Website
A parallax website employs a visually striking effect in which background images move at varied speeds in response to user scrolling, enhancing visual engagement.
Example Code:
<!DOCTYPE html>
<html>
<head>
<title>Parallax Experience</title>
<style>
.parallax {
background-image: url('background.jpg');
height: 500px;
background-attachment: fixed;
background-position: center;
background-repeat: no-repeat;
background-size: cover;
}
</style>
</head>
<body>
<div class="parallax"></div>
</body>
</html>
5. Blog Website
A blog website facilitates content publication, providing a structured format for text, images, and hyperlinks.
Example Code:
<!DOCTYPE html>
<html>
<head>
<title>Scholarly Insights</title>
</head>
<body>
<h1>Academic Perspectives</h1>
<article>
<h2>First Entry</h2>
<p>Exploring the intersection of technology and human cognition.</p>
</article>
</body>
</html>
6. Photo Gallery Website
A photo gallery website presents images in a structured and interactive format, utilizing grids and dynamic layouts.
Example Code:
<!DOCTYPE html>
<html>
<head>
<title>Visual Archive</title>
</head>
<body>
<h1>Photography Collection</h1>
<div>
<img src="image1.jpg" alt="Scenic View">
<img src="image2.jpg" alt="Urban Landscape">
</div>
</body>
</html>
7. Recipe Book Website
A recipe book website catalogs culinary instructions, ingredient lists, and illustrative imagery in a structured format.
Example Code:
<!DOCTYPE html>
<html>
<head>
<title>Gastronomic Chronicles</title>
</head>
<body>
<h1>Gourmet Chocolate Cake</h1>
<ul>
<li>Organic Flour</li>
<li>Unrefined Sugar</li>
<li>Free-range Eggs</li>
</ul>
</body>
</html>
8. YouTube Clone
A YouTube clone replicates fundamental video-sharing functionalities, enabling video playback and user interaction.
Example Code:
<!DOCTYPE html>
<html>
<head>
<title>Media Streaming Hub</title>
</head>
<body>
<h1>Featured Video</h1>
<video controls>
<source src="media.mp4" type="video/mp4">
</video>
</body>
</html>
9. To-do List Application
A to-do list application enables efficient task management through interactive functionalities such as task addition, deletion, and completion tracking.
Example Code:
<!DOCTYPE html>
<html>
<head>
<title>Task Management System</title>
<script>
function addTask() {
let task = document.createElement("li");
task.innerText = document.getElementById("taskInput").value;
document.getElementById("taskList").appendChild(task);
}
</script>
</head>
<body>
<h1>Organized Workflow</h1>
<input type="text" id="taskInput">
<button onclick="addTask()">Add Task</button>
<ul id="taskList"></ul>
</body>
</html>
Conclustions
By engaging with these HTML-based projects, developers can cultivate proficiency in structuring web applications, implementing responsive designs, and optimizing user experience. These projects serve as an excellent foundation for advancing technical expertise and professional portfolio development.