TypeORM with NestJS: A Beginner's Guide to Database Integration
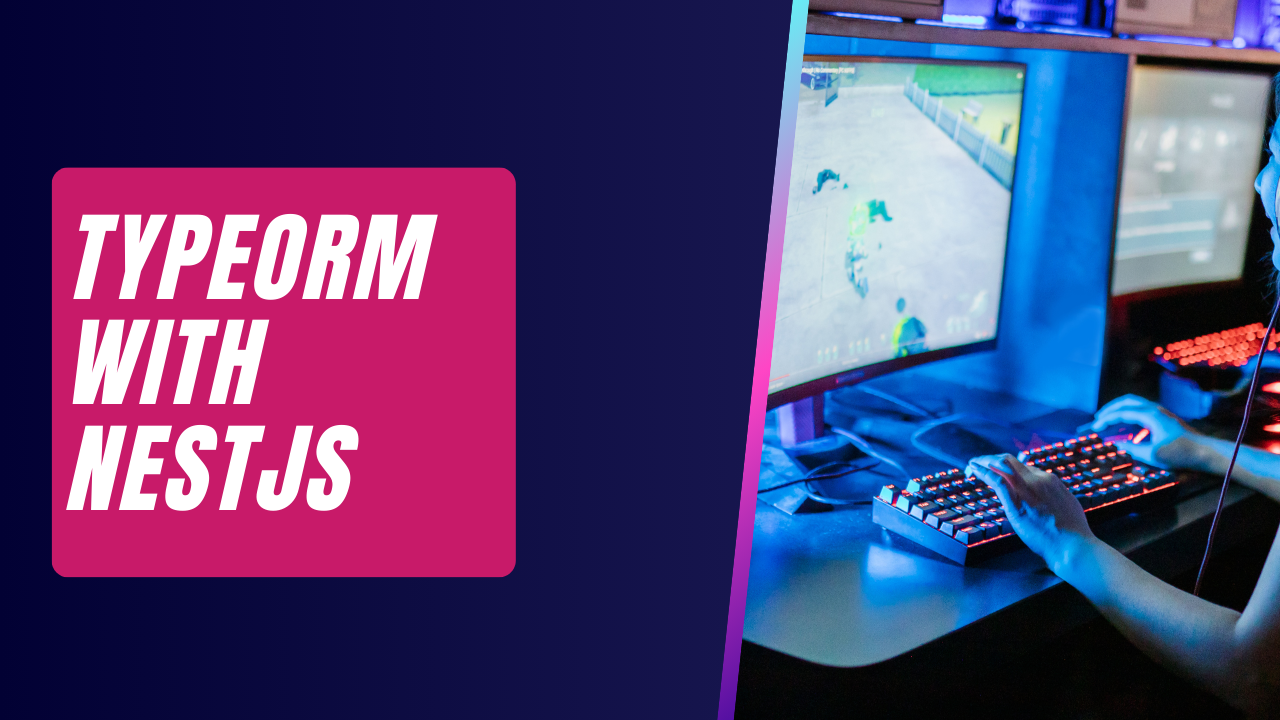
Integrating TypeORM with NestJS establishes a sophisticated framework for architecting scalable, database-centric applications.
This discourse meticulously explores the complete implementation cycle, from foundational setup to intricate functionalities, providing an exhaustive roadmap for proficient developers leveraging TypeORM within NestJS.
Rationale for Employing TypeORM with NestJS
TypeORM, as a powerful Object-Relational Mapper (ORM), facilitates seamless interaction with relational databases through TypeScript. When coupled with NestJS, it delivers several technical advantages:
- Enhanced Type Safety: Guarantees compile-time validation of database queries, minimizing runtime errors.
- Decorator-Oriented Entity Definition: Streamlines schema modeling through intuitive, metadata-driven class decorators.
- Database Abstraction: Ensures compatibility with multiple database management systems, including PostgreSQL, MySQL, and SQLite.
- Migration and Schema Synchronization Capabilities: Supports versioned database schema evolution with built-in migration tools.
- Optimized Scalability: Ideal for distributed microservices architectures requiring modular persistence layers.
Configuring TypeORM in a NestJS Environment
Prerequisites
- Node.js (version 18+ recommended)
- npm or yarn package manager
- Proficiency in TypeScript
- A relational database (PostgreSQL/MySQL/SQLite)
1. Establishing a NestJS Project
npm install -g @nestjs/cli
nest new typeorm-nest-project
cd typeorm-nest-project
2. Installing TypeORM Dependencies
For PostgreSQL:
npm install @nestjs/typeorm typeorm pg
For MySQL:
npm install @nestjs/typeorm typeorm mysql2
3. Database Configuration
Create ormconfig.json
:
{
"type": "postgres",
"host": "localhost",
"port": 5432,
"username": "postgres",
"password": "secret",
"database": "nestjs_db",
"entities": ["dist/**/*.entity{.ts,.js}"],
"synchronize": true
}
Modify app.module.ts
:
import { TypeOrmModule } from '@nestjs/typeorm';
@Module({
imports: [TypeOrmModule.forRoot()],
})
export class AppModule {}
Entity Construction and Relational Mapping
Defining Entity Schemas
// user.entity.ts
import { Entity, PrimaryGeneratedColumn, Column } from 'typeorm';
@Entity()
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
@Column({ unique: true })
email: string;
@Column()
password: string;
}
Implementing One-to-Many Relationships
// post.entity.ts
import { Entity, PrimaryGeneratedColumn, Column, ManyToOne } from 'typeorm';
import { User } from './user.entity';
@Entity()
export class Post {
@PrimaryGeneratedColumn()
id: number;
@Column()
title: string;
@ManyToOne(() => User, user => user.posts)
author: User;
}
// Augmenting the User entity
import { OneToMany } from 'typeorm';
import { Post } from './post.entity';
@OneToMany(() => Post, post => post.author)
posts: Post[];
Implementing the Repository Pattern
Configuring the Users Module
// users.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { User } from './user.entity';
import { UsersService } from './users.service';
@Module({
imports: [TypeOrmModule.forFeature([User])],
providers: [UsersService],
})
export class UsersModule {}
Service Layer Implementation
// users.service.ts
import { Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { User } from './user.entity';
import { CreateUserDto } from './dto/create-user.dto';
@Injectable()
export class UsersService {
constructor(
@InjectRepository(User)
private usersRepository: Repository<User>
) {}
async create(userData: CreateUserDto): Promise<User> {
const newUser = this.usersRepository.create(userData);
return this.usersRepository.save(newUser);
}
async findAll(): Promise<User[]> {
return this.usersRepository.find();
}
}
Database Schema Migrations
Executing Migration Commands
typeorm migration:create -n CreateUsersTable
typeorm migration:run
typeorm migration:revert
Example Migration Definition
import { MigrationInterface, QueryRunner } from 'typeorm';
export class CreateUsersTable1700000000000 implements MigrationInterface {
public async up(queryRunner: QueryRunner): Promise<void> {
await queryRunner.query(`
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name VARCHAR(255),
email VARCHAR(255) UNIQUE,
password VARCHAR(255)
)
`);
}
public async down(queryRunner: QueryRunner): Promise<void> {
await queryRunner.query(`DROP TABLE users`);
}
}
Performance Optimization Strategies
- Efficient Query Design: Utilize indexed queries and avoid unnecessary joins.
- Lazy Loading Strategies: Optimize entity fetching to reduce database load.
- Query Caching: Implement repository-level caching for recurrent queries.
- Connection Pooling: Configure database connection pools to enhance throughput.
Architectural Best Practices
- Data Validation: Enforce schema integrity using
class-validator
decorators within DTOs. - Robust Error Handling: Implement global exception filters for standardized error responses.
- Unit and Integration Testing: Employ Jest for comprehensive service and repository testing.
- Security Enhancements: Utilize bcrypt for password hashing and implement access control mechanisms.
- API Documentation: Leverage OpenAPI/Swagger for well-defined API specifications.
// DTO with Validation
import { IsEmail, IsString } from 'class-validator';
export class CreateUserDto {
@IsString()
name: string;
@IsEmail()
email: string;
}
Conclusion
The integration of TypeORM with NestJS provides a powerful, structured approach to database management within modern backend applications.
By adhering to best practices, implementing robust entity relationships, and leveraging advanced query optimizations, developers can construct scalable, efficient, and maintainable services.
This guide serves as a foundational reference, equipping engineers with the knowledge to harness the full potential of TypeORM within the NestJS ecosystem.